Understanding `some View` in SwiftUI
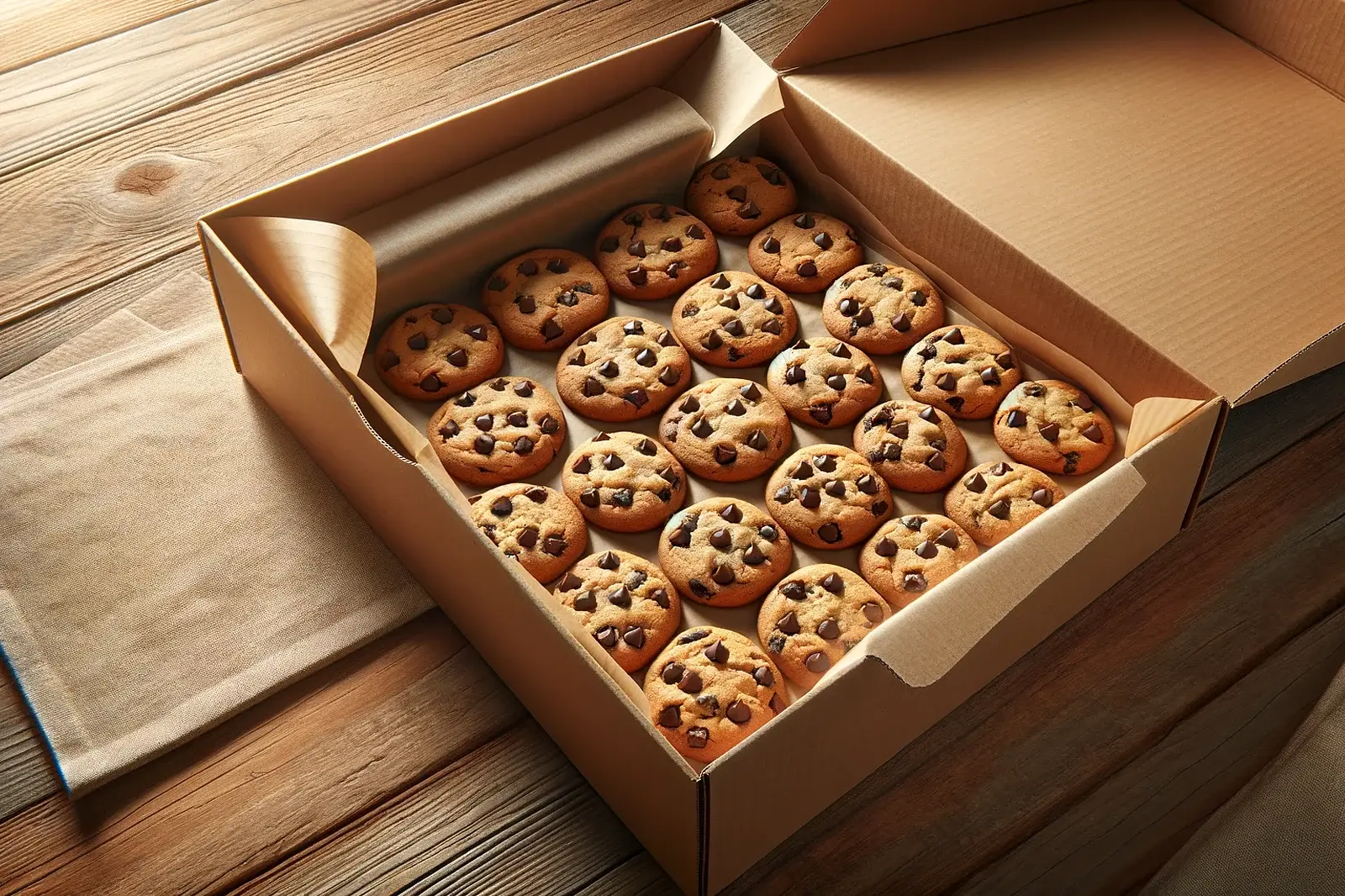
If you're jumping into SwiftUI, you will see some View
immediately, and it's a somewhat complicated topic to understand so early in your journey. Thankfully, Swift 5.7 made a minor but helpful tweak to the keywords that helped clarify what's happening.
This post will help you understand the differences between the some
and any
keywords when used with protocols and why conforming to the View
protocol was a bit harder to understand before April 2022.
What is some View
?
If you start a new iOS project in Xcode, you will see this bit in ContentView.swift:
struct ContentView: View {
var body: some View { // <- what is this?
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundStyle(.tint)
Text("Hello, world!")
}
.padding()
}
}
You'll get familiar with this code because it's found in one of the first files you'll touch in every project! We're focused on the line with var body: some View
. SwiftUI requires the some
keyword to help the View
protocol understand the associatedtype
it will deal with. The good news is you don't really need to know what's going on inside the View
protocol; you just need to understand that behind the scenes, SwiftUI needs specificity for performance reasons.
It helps to speak what's happening out loud, "I am defining a computed property called body
, and it conforms to some View
." We are "promising" SwiftUI that we're giving it a required layout, but we refuse to explain it. It's a good deal on our part! Make that "promise" using some,
and you're good to go.
Clarifications in Swift 5.7
I was making my first pass through Swift in late 2021, which meant I would have been using Swift 5.5.x. Then, not using the some
keyword and just writing var body: View
in this scenario would have produced an error:
protocol can only be used as a generic constraint because it has Self or associated type requirements
Honestly, that's a pretty cryptic error if you're just getting your feet wet. The Swift team must have thought so, too, because around April 2022, they released Swift 5.7 and, along with it, a new keyword requirement called any
.
Trying to run the same var body: View
line of code now produces a new error:
Use of protocol 'View' as a type must be written 'any View'
Interesting! But what is any
all about?
The any
keyword
The any
keyword tells the compiler that you're planning on giving it a box, and whatever is inside that box will conform to the specified protocol. They even call it a boxed protocol type. Doing this has the potential for added flexibility but at the cost of performance. Swift has to do more work to look inside the box and sort things out because it doesn't know exactly what's in there. When we use some
, we gain optimization because it knows the type in advance... there's no box!
The addition of the any
keyword does a great job of helping us understand some
. If I say I'll give you "some cookies," you would assume you'll get a type of cookie you can identify: chocolate chip. If I say I'll give you "any cookies," you'll know you're getting a cookie, but you couldn't be sure of the type.
tl;dr
Using some View
helps us keep things fast, and fast is what we want when we're dealing with rendering our views. Don't be too concerned about the nitty-gritty details if you're in the early days of your iOS/Swift journey. Just know that you're promising SwiftUI a layout that conforms to the View
protocol and getting to walk away happy knowing the compiler will figure out the rest.